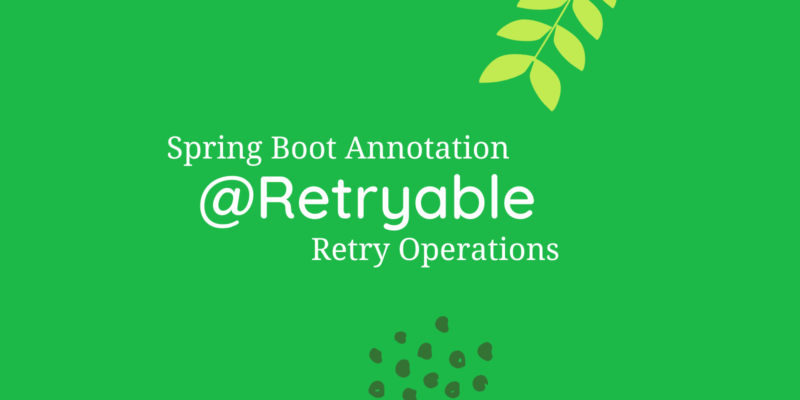
How to use @Retryable annotation in a spring boot application
The @Retryable
annotation in Spring is a powerful mechanism that allows developers to handle transient failures in a graceful and customisable manner. It is part of the Spring Retry project, which provides support for retrying operations. In this post we will discuss how to use @Retryable annotation in a spring boot application.
Overview
The @Retryable
annotation is used in Spring applications to indicate that a particular method should be retried in case of failure. This annotation is particularly useful when dealing with operations that may fail due to transient issues, such as network glitches or temporary unavailability of resources.
Dependency
To use @Retryable
, you need to include the Spring Retry dependency in your project. If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>org.springframework.retry</groupId>
<artifactId>spring-retry</artifactId>
<version>1.3.1</version> <!-- Use the latest version available -->
</dependency>
Enabling Retry Support with @EnableRetry
The @EnableRetry
annotation is used to enable support for the @Retryable
annotation and other retry-related features in a Spring application. It is typically placed at the configuration level, such as on a @Configuration
class or in the main application class annotated with @SpringBootApplication
. By using @EnableRetry
, you indicate to Spring that you want to activate the retry functionality provided by the Spring Retry project.
In the Main Application Class (e.g., Spring Boot Application)
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.retry.annotation.EnableRetry;
@SpringBootApplication
@EnableRetry
public class YourSpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(YourSpringBootApplication.class, args);
}
}
In a Separate Configuration Class (e.g., RetryConfig)
import org.springframework.context.annotation.Configuration;
import org.springframework.retry.annotation.EnableRetry;
@Configuration
@EnableRetry
public class RetryConfig {
// Configuration beans and other settings
}
When @EnableRetry
is present in your application, Spring will recognize and process methods annotated with @Retryable
. Without @EnableRetry
, the @Retryable
annotation won’t have any effect, and the retry functionality won’t be enabled.
Basic Example
Let’s start with a simple example. Assume you have a service method that interacts with an external service, and you want to retry the operation in case of a RuntimeException
. Here’s how you can use @Retryable
:
import org.springframework.retry.annotation.Retryable;
@Service
public class MyService {
@Retryable(RuntimeException.class)
public void performOperation() {
// Your code that might throw a RuntimeException
}
}
In this example, if a RuntimeException
is thrown during the execution of performOperation()
, Spring will automatically retry the method according to the default retry configuration.
Working of @Retryable
Configuration
@Retryable
provides several attributes that allow you to customize its behavior:
value
(aliases:exception
,exceptions
): Specifies the exception or exceptions that should trigger a retry. You can provide a single exception class or an array of classes.maxAttempts
: Specifies the maximum number of retry attempts. The default is 3.backoff
: Specifies the backoff policy for waiting between retry attempts. It accepts an array of@Backoff
annotations, each defining a different backoff strategy.
Backoff Strategies
The @Backoff
annotation is used to define the backoff policy for waiting between retry attempts. It has two attributes:
delay
: The initial delay before the first retry, in milliseconds. The default is 1000 milliseconds (1 second).maxDelay
: The maximum delay between retries, in milliseconds. The default is 0, which means no maximum delay.
Here’s an example of using @Retryable
with custom backoff:
import org.springframework.retry.annotation.Backoff;
import org.springframework.retry.annotation.Retryable;
@Service
public class MyService {
@Retryable(value = RuntimeException.class, maxAttempts = 5, backoff = @Backoff(delay = 500, maxDelay = 2000))
public void performOperation() {
// Your code that might throw a RuntimeException
}
}
In this example, the method will be retried up to 5 times with an initial delay of 500 milliseconds and a maximum delay of 2000 milliseconds between retries.
Advanced Features
Customizing Retry Policies
In addition to the basic @Retryable
annotation, Spring Retry provides more advanced features for customizing retry policies. You can define your own RetryPolicy
and BackOffPolicy
and use them in conjunction with @Retryable
.
Here’s an example of using a custom RetryPolicy
:
import org.springframework.retry.annotation.Retryable;
import org.springframework.retry.policy.SimpleRetryPolicy;
@Service
public class MyService {
@Retryable(value = RuntimeException.class, retryPolicy = "customRetryPolicy")
public void performOperation() {
// Your code that might throw a RuntimeException
}
@Bean
public RetryPolicy customRetryPolicy() {
return new SimpleRetryPolicy(5); // Retry up to 5 times
}
}
In this example, the customRetryPolicy
method creates a SimpleRetryPolicy
with a maximum of 5 retry attempts, and the performOperation
method uses this custom policy.
Conditionally Retrying Methods
You can use the @Retryable
annotation in combination with a RetryCondition
to conditionally retry a method based on certain criteria. Here’s an example:
import org.springframework.retry.annotation.Retryable;
import org.springframework.retry.condition.ContextRetryCondition;
@Service
public class MyService {
@Retryable(value = RuntimeException.class, condition = "retryCondition")
public void performOperation() {
// Your code that might throw a RuntimeException
}
@Bean
public ContextRetryCondition retryCondition() {
return new ContextRetryCondition();
}
}
In this example, the retryCondition
method creates a ContextRetryCondition
, and the performOperation
method uses this condition to determine whether to retry or not.
The @Retryable
annotation in Spring provides a convenient and flexible way to handle transient failures in your applications. Whether you need simple retries with default configurations or advanced customization with custom retry and backoff policies, Spring Retry has you covered. By using @Retryable
wisely, you can improve the resilience of your applications in the face of intermittent issues, making them more robust and reliable.
You can read the documentation here – @Retryable Annotation
In the last post we had discussed How to use @Conditional Annotation in Spring Boot Application.
Happy Learning!
Subscribe to the BitsToGigs Newsletter