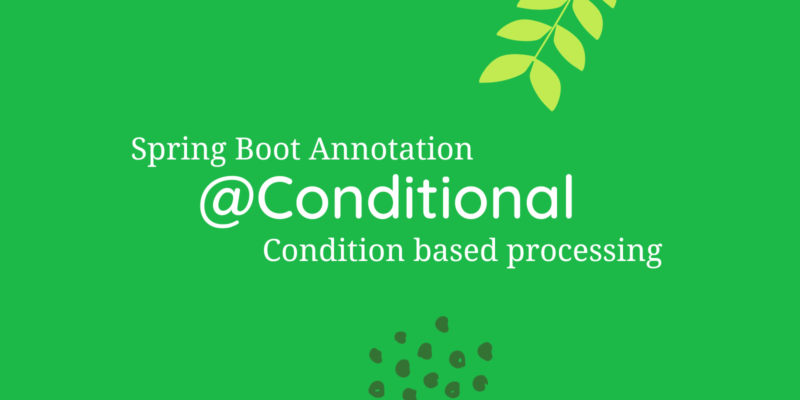
How to use @Conditional Annotation in a Spring Boot Application
The @Conditional
annotation in Spring is a powerful mechanism that allows you to conditionally enable or disable the processing of a bean or a configuration class based on certain conditions. This annotation provides a way to dynamically control the bean registration process in a Spring application. In this post we will discuss how to use @Conditional Annotation in Spring Boot App.
Introduction to @Conditional Annotation
In a Spring application, beans are typically defined using annotations like @Component
, @Service
, @Repository
, or @Configuration
. However, there might be scenarios where you want to conditionally register a bean based on certain conditions such as the presence of a specific property, the existence of a certain class in the classpath, or any custom condition you define.
This is where the @Conditional
annotation comes into play. By placing this annotation on a bean definition or configuration class, you can specify a condition that must be satisfied for the bean to be registered.
How to use @conditional annotation
The @Conditional
annotation can be used in conjunction with other annotations to conditionally enable or disable beans. Let’s see how to use @conditional annotation with a example:
@Configuration
@Conditional(MyCondition.class)
public class MyConditionalConfiguration {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
In this example, the MyBean
bean will only be registered if the condition specified by MyCondition
is satisfied. The MyCondition
class should implement the Condition
interface, which is part of the Spring framework.
Common Conditions
Spring provides several built-in conditions that you can use out of the box. Some of the commonly used ones include:
@ConditionalOnClass
This condition checks if the specified classes are present in the classpath.
@Configuration
@ConditionalOnClass(name = "com.example.MyClass")
public class MyClassConfiguration {
// Bean definitions go here
}
@ConditionalOnProperty
This condition checks if the specified property is present in the environment
@Configuration
@ConditionalOnProperty(name = "my.property")
public class MyPropertyConfiguration {
// Bean definitions go here
}§
@ConditionalOnMissingBean
This condition checks if a bean of the specified type is not already present.
@Configuration
@ConditionalOnMissingBean(MyBean.class)
public class MyBeanConfiguration {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
@ConditionalOnExpression
This condition allows you to use SpEL (Spring Expression Language) expressions to determine whether the condition is met.
@Configuration
@ConditionalOnExpression("${my.condition}")
public class MyExpressionConfiguration {
// Bean definitions go here
}
These conditions provide a flexible way to control the registration of beans based on various criteria.
Custom Conditions
In addition to the built-in conditions, you can create your own custom conditions by implementing the Condition
interface. Here’s a simple example:
public class MyCustomCondition implements Condition {
@Override
public boolean matches(ConditionContext context, AnnotatedTypeMetadata metadata) {
// Your custom condition logic goes here
return true; // Return true if the condition is met, false otherwise
}
}
You can then use your custom condition in a configuration class:
@Configuration
@Conditional(MyCustomCondition.class)
public class MyCustomConfiguration {
// Bean definitions go here
}
Real World Example
Let’s consider a real-world example where you want to conditionally enable a caching mechanism only if a certain property is set in the application’s configuration:
@Configuration
@ConditionalOnProperty(name = "cache.enabled", havingValue = "true")
public class CacheConfiguration {
@Bean
public CacheManager cacheManager() {
// Create and configure the cache manager
return new MyCacheManager();
}
}
In this example, the CacheConfiguration
class will only be processed and the CacheManager
bean will be registered if the property cache.enabled
is set to true
in the application’s properties or YAML file.
The @Conditional
annotation in Spring is a powerful tool that allows you to conditionally enable or disable beans based on various conditions and in this post we discussed how to use @Conditional annotation in different scenarios. Whether you need to check for the presence of a class, the existence of a property, or any other custom condition, the @Conditional
annotation provides a flexible and extensible mechanism for controlling the bean registration process in your Spring applications. Understanding how to use and create custom conditions can greatly enhance the flexibility and configurability of your Spring-based applications.
You can read the documentation on @conditional annotation at the following link – @Conditional Annotation
Out last post was on Spring Boot JUnit Tests – The Most common challenges developers see
Happy Learning and go from Bits to Gigs!
Subscribe to the BitsToGigs Newsletter