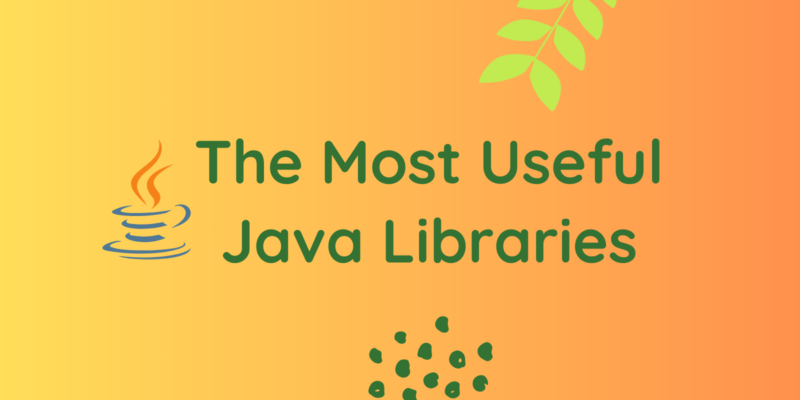
The Most Useful Java Libraries All Developers Must Know
Java is a versatile programming language widely used in software development, and engineers often rely on various libraries to enhance their productivity and streamline development processes. In this BitsToGigs post we will explore The Most Useful Java Libraries All Developers Must Know.
Lombok
Lombok, short for “Project Lombok,” is a Java library that operates during the compilation phase to reduce the verbosity of Java code. It achieves this by introducing annotations that automatically generate common boilerplate code, such as getters, setters, constructors, and more. By doing so, Lombok helps developers write concise, readable, and maintainable code without sacrificing functionality.
You can read more here: All You need to know about Lombok Library for Java Development
Apache Commons Lang
Apache Commons Lang provides a set of utility classes and methods for common programming tasks, such as string manipulation, array handling, and more. It simplifies many routine tasks, making code more concise and readable.
Example:
import org.apache.commons.lang3.StringUtils;
public class CommonLangExample {
public static void main(String[] args) {
String text = "Hello, Commons Lang!";
// Check if the string is empty or null
if (StringUtils.isNotBlank(text)) {
System.out.println(StringUtils.upperCase(text));
}
}
}
Guava
Developed by Google, Guava is a comprehensive set of libraries that offer a wide range of functionalities, including collections, caching, concurrency, and more. It enhances the capabilities of the Java Standard Library and promotes cleaner, more efficient code.
Example:
import com.google.common.collect.Lists;
public class GuavaExample {
public static void main(String[] args) {
// Create an immutable list
var immutableList = Lists.immutable.of("Guava", "Library", "Example");
// Print the elements
immutableList.forEach(System.out::println);
}
}
Jackson
Jackson is a high-performance JSON processor for Java. It provides a set of tools for parsing JSON data into Java objects and vice versa. Jackson is widely used in web applications and microservices for handling JSON serialization and deserialization.
Example:
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
// Convert object to JSON
String jsonString = objectMapper.writeValueAsString(new YourObject());
// Convert JSON to object
YourObject yourObject = objectMapper.readValue(jsonString, YourObject.class);
}
}
JUnit
JUnit is a popular testing framework for Java that simplifies the process of writing and running unit tests. It provides annotations for test methods, assertions for verifying expected results, and test runners for executing tests. JUnit is an essential tool for achieving code reliability.
Example:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class JUnitExample {
@Test
public void testAddition() {
int result = Calculator.add(2, 3);
assertEquals(5, result);
}
}
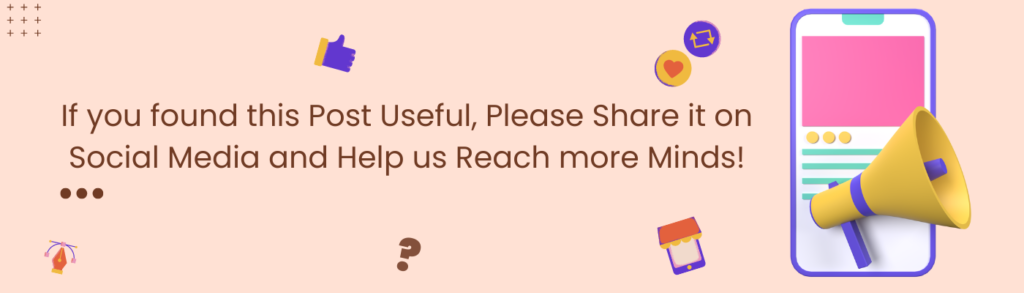
Log4j
Log4j is a robust logging library that allows developers to log messages from applications. It provides various logging levels, configurable output targets, and is highly customizable. Log4j is crucial for monitoring and debugging applications.
Example
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Log4jExample {
private static final Logger LOGGER = LogManager.getLogger(Log4jExample.class);
public static void main(String[] args) {
LOGGER.info("Hello, Log4j!");
}
}
Spring Framework
Spring is a comprehensive framework for Java development, providing support for building enterprise-level applications. It includes modules for dependency injection, data access, web development, and more. Spring simplifies complex tasks and promotes best practices in software design.
Example: (Spring is often used across multiple modules; here’s a simple example for context initialization)
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class SpringExample {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
YourBean yourBean = context.getBean(YourBean.class);
yourBean.doSomething();
}
}
Hibernate
Hibernate is a powerful and flexible ORM (Object-Relational Mapping) framework for Java, simplifying database interactions.
Example: (A basic example showing entity mapping and retrieval)
import org.hibernate.Session;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class HibernateExample {
public static void main(String[] args) {
// Initialize Hibernate
SessionFactory factory = new Configuration().configure("hibernate.cfg.xml").buildSessionFactory();
// Create a session
Session session = factory.getCurrentSession();
try {
// Begin a transaction
session.beginTransaction();
// Perform database operations (e.g., save, update, query)
// Commit the transaction
session.getTransaction().commit();
} finally {
// Close the session and factory
session.close();
factory.close();
}
}
}
In the last BitsToGigs.com article we had discussed about API development in Spring Boot. You can read that here – How to achieve Dependency Injection in a Spring Boot App
Happy Learning!
Subscribe to the BitsToGigs Newsletter