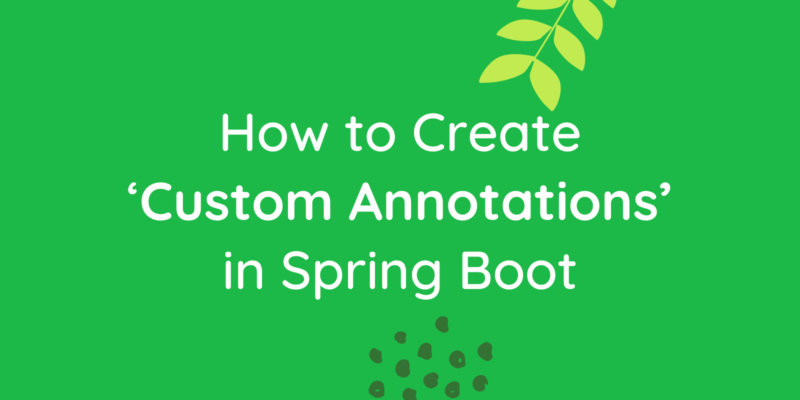
How to Create Custom Annotations in a Spring Boot App
Spring Boot, a popular framework for building Java-based enterprise applications, provides a powerful and flexible way to develop applications quickly and efficiently. One of the key features of Spring Boot is its support for annotations, which simplify the configuration and development process. In this article, we’ll explore how to create custom annotations in a Spring Boot application, including detailed examples, use cases, and best practices.
Understanding Annotations in Spring Boot
Annotations in Spring Boot provide metadata to the Spring container, allowing it to understand how to configure and manage beans, components, and other elements of your application. Spring Boot comes with a rich set of built-in annotations, such as @RestController
, @Service
, and @Autowired
, which simplify common development tasks.
Creating custom annotations allows you to encapsulate specific behaviors or configurations and reuse them across your codebase. This can enhance code readability, maintainability, and reduce duplication.
Anatomy of a Custom Annotation
Before diving into examples, let’s understand the basic structure of a custom annotation in Java. A custom annotation is defined using the @interface
keyword, followed by the annotation name. It can include attributes, which act as parameters for the annotation.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface CustomAnnotation {
String value() default "default value";
}
In this example:
@Retention(RetentionPolicy.RUNTIME)
: Specifies that the annotation should be retained at runtime, allowing reflection to access it.@Target(ElementType.METHOD)
: Specifies where the annotation can be applied—in this case, to methods.
Creating a Simple Custom Annotation
Let’s create a simple custom annotation called LogExecutionTime
, which logs the execution time of a method.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface LogExecutionTime {
}
Now, let’s apply this annotation to a method in a Spring Boot service class.
@Service
public class MyService {
@LogExecutionTime
public void performTask() {
// Method logic
}
}
Implementing a Custom Aspect for Annotation Processing
To make our custom annotation LogExecutionTime
functional, we need to create an aspect that intercepts method calls and logs the execution time. In Spring, aspects are used to modularize cross-cutting concerns.
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
import java.util.Arrays;
@Aspect
@Component
public class LogExecutionTimeAspect {
@Around("@annotation(LogExecutionTime)")
public Object logExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long startTime = System.currentTimeMillis();
Object result = joinPoint.proceed();
long endTime = System.currentTimeMillis();
long executionTime = endTime - startTime;
System.out.println(
String.format("Method %s execution time: %d ms",
joinPoint.getSignature(), executionTime));
return result;
}
}
In this aspect:
@Aspect
: Declares the class as an aspect.@Around("@annotation(LogExecutionTime)")
: Specifies that the advice (code to be executed) should run around methods annotated with@LogExecutionTime
.
Real-World Use Case: Transaction Management
Let’s explore a real-world use case for custom annotations by creating an annotation for transaction management. We’ll create an annotation called @TransactionalTask
that ensures a method is executed within a transactional context.
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface TransactionalTask {
String value() default "default-transaction-manager";
}
Now, let’s create an aspect to handle the transaction management.
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
import org.springframework.transaction.annotation.Transactional;
@Aspect
@Component
public class TransactionalTaskAspect {
@Around("@annotation(transactionalTask)")
public Object manageTransaction(ProceedingJoinPoint joinPoint, TransactionalTask transactionalTask) throws Throwable {
String transactionManager = transactionalTask.value();
TransactionTemplate transactionTemplate = new TransactionTemplate(
new JtaTransactionManagerFactoryBean(transactionManager).getObject());
return transactionTemplate.execute(status -> {
try {
return joinPoint.proceed();
} catch (Throwable throwable) {
throw new RuntimeException(throwable);
}
});
}
}
In this example, we use Spring’s @Transactional
annotation internally to manage the transaction.
In conclusion, creating custom annotations in Spring Boot allows you to encapsulate and reuse common behaviours and configurations across your codebase. In this article, we explored the basics of custom annotations, demonstrated how to create a simple custom annotation, and implemented a real-world use case with transaction management. As you integrate custom annotations into your projects, remember to adhere to best practices, keep your codebase modular, and leverage annotations to enhance the readability and maintainability of your code.
In the last BitsToGigs post we had discussed MongoDB CRUD operations in a spring boot app simplified
Happy Learning!
Subscribe to the BitsToGigs Newsletter