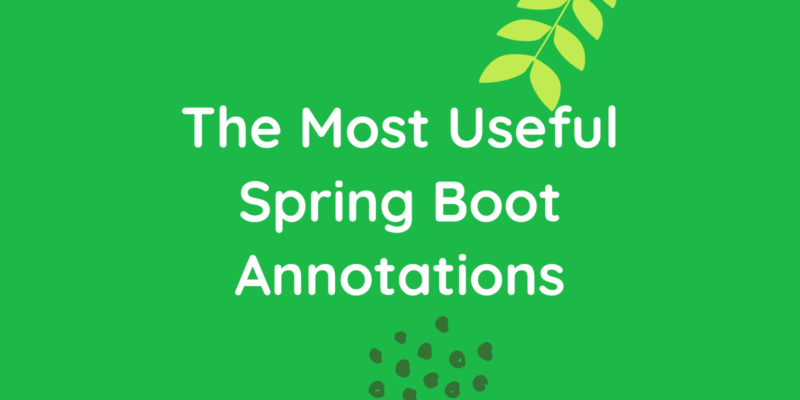
The Most Useful Spring Boot Annotations all Devs Must Know
Spring Boot is a popular framework for building Java-based enterprise applications. Annotations play a crucial role in simplifying the configuration and development process in Spring Boot. In this article, we’ll explore different categories of the most useful Spring Boot annotations, providing descriptions and example code for each category.
1. Configuration Annotations
@SpringBootApplication
This annotation is used to mark the main class of a Spring Boot application. It combines three other annotations: @Configuration
, @EnableAutoConfiguration
, and @ComponentScan
. It essentially bootstraps and auto-configures a Spring application.
@SpringBootApplication
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
@Configuration
This annotation indicates that a class declares one or more @Bean
methods. It is often used in combination with @ComponentScan
to enable component scanning for Spring-managed beans.
@Configuration
public class MyConfiguration {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
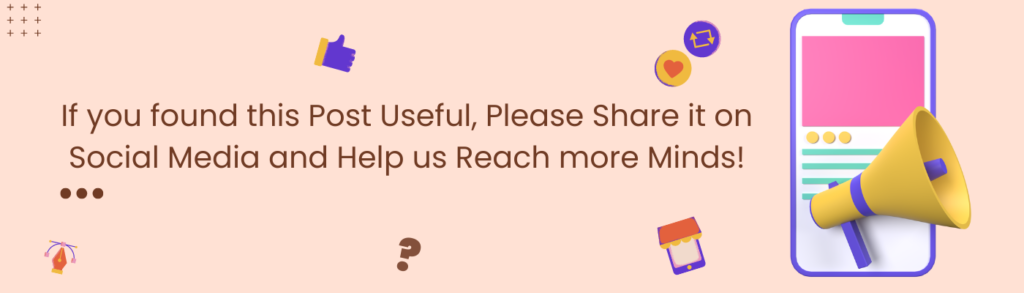
2. Component Annotations
@Component
This annotation is a generic stereotype annotation for any Spring-managed component. It indicates that the class is a Spring component, and the Spring container will manage its lifecycle.
@Component
public class MyComponent {
// Class implementation
}
@Service
, @Repository
, @Controller
These are specializations of @Component
and are used to indicate specific types of Spring-managed components: services, repositories, and controllers, respectively.
@Service
public class MyService {
// Service implementation
}
@Repository
public class MyRepository {
// Repository implementation
}
@Controller
public class MyController {
// Controller implementation
}
3. Dependency Injection Annotations
@Autowired
This annotation is used to automatically inject a dependency into a Spring bean. It can be applied to fields, setter methods, or constructors.
@Service
public class MyService {
private final MyRepository myRepository;
@Autowired
public MyService(MyRepository myRepository) {
this.myRepository = myRepository;
}
// Service methods using myRepository
}
4. Aspect-Oriented Programming (AOP) Annotations
@Aspect
This annotation is used to declare a class as an aspect, allowing it to define advice (methods) and pointcuts.
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.service.*.*(..))")
public void beforeServiceMethod() {
// Advice implementation
}
}
5. Data Access Annotations
@Entity
, @Table
, @Column
These annotations are used for defining JPA entities, specifying table and column details.
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "username")
private String username;
// Other fields and methods
}
@Repository
This annotation is used to indicate that a class is a Spring Data repository.
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
// Custom query methods
}
6. Web Annotations
@Controller
, @RestController
These annotations are used to define Spring MVC controllers. @RestController
is a specialization of @Controller
that simplifies the creation of RESTful web services.
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping
public List<User> getAllUsers() {
// Retrieve and return a list of users
}
}
@RequestMapping
, @GetMapping
, @PostMapping
These annotations are used to map HTTP requests to specific handler methods.
@RestController
@RequestMapping("/api/greetings")
public class GreetingController {
@GetMapping
public String getGreeting() {
return "Hello, World!";
}
@PostMapping
public String postGreeting() {
return "Greeting posted!";
}
}
These are just a few examples of the many annotations available in Spring Boot. Annotations simplify the development process by providing a declarative way to configure and manage various aspects of a Spring application. Understanding and using these annotations effectively can significantly enhance the productivity and maintainability of Spring Boot projects.
7. RESTful Web Services Annotations
@PathVariable
This annotation is used to extract values from the URI path and bind them to method parameters.
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/{userId}")
public ResponseEntity<User> getUserById(@PathVariable Long userId) {
// Retrieve user by ID and return as ResponseEntity
}
}
@RequestBody
This annotation is used to bind the HTTP request body to a method parameter.
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
// Save the user and return as ResponseEntity
}
}
8. Validation Annotations
@Valid
This annotation is used in conjunction with @RequestBody
to trigger validation of the request body.
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping
public ResponseEntity<User> createUser(@Valid @RequestBody User user) {
// Save the validated user and return as ResponseEntity
}
}
@NotNull
, @NotBlank
, @Size
These annotations are used to specify validation constraints on fields.
public class User {
@NotNull
private Long id;
@NotBlank
@Size(min = 3, max = 50)
private String username;
// Other fields and methods
}
9. Security Annotations
@Secured
, @PreAuthorize
, @PostAuthorize
These annotations are used to define method-level security.
@RestController
@RequestMapping("/api/admin")
public class AdminController {
@Secured("ROLE_ADMIN")
@GetMapping("/all-users")
public List<User> getAllUsers() {
// Retrieve and return a list of users
}
@PreAuthorize("hasRole('ADMIN') and hasIpAddress('192.168.0.1')")
@PostMapping("/create-user")
public ResponseEntity<User> createUser(@RequestBody User user) {
// Save the user and return as ResponseEntity
}
}
10. Scheduled Tasks Annotations
@Scheduled
This annotation is used to schedule tasks to be executed at fixed intervals.
@Component
public class MyScheduledTask {
@Scheduled(fixedRate = 5000)
public void performTask() {
// Task implementation
}
}
11. Caching Annotations
@EnableCaching
, @Cacheable
, @CacheEvict
These annotations are used for caching in Spring applications.
@EnableCaching
public class MyCacheConfig {
// Cache configuration
}
@Service
public class MyService {
@Cacheable("users")
public User getUserById(Long userId) {
// Method implementation
}
@CacheEvict("users")
public void evictUserCache(Long userId) {
// Method implementation
}
}
12. Testing Annotations
@SpringBootTest
, @RunWith(SpringRunner.class)
These annotations are used for testing Spring Boot applications.
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testGetUserById() {
// Test implementation
}
}
13. Transaction Management Annotations
@Transactional
This annotation is used to define the scope of a transaction. It can be applied at the class or method level.
@Service
public class MyTransactionalService {
@Transactional
public void performTransaction() {
// Transactional method implementation
}
}
@EnableTransactionManagement
This annotation is used at the configuration class to enable Spring’s annotation-driven transaction management.
@Configuration
@EnableTransactionManagement
public class MyTransactionConfig {
// Transaction management configuration
}
14. Message-Driven Annotations
@EnableJms
, @JmsListener
These annotations are used for setting up and listening to JMS (Java Message Service) queues or topics.
@Configuration
@EnableJms
public class JmsConfig {
// JMS configuration
}
@Service
public class JmsMessageListener {
@JmsListener(destination = "myQueue")
public void handleMessage(String message) {
// Process the JMS message
}
}
15. Internationalization (i18n) Annotations
@EnableMessageSource
, @ConfigurationProperties
These annotations are used for setting up internationalization in a Spring Boot application.
@Configuration
@EnableMessageSource
public class MessageSourceConfig {
// Message source configuration
}
@Component
@ConfigurationProperties(prefix = "myapp.messages")
public class MyAppMessages {
private String welcomeMessage;
// Getter and setter methods
}
16. Swagger Annotations
@EnableSwagger2
, @Api
, @ApiOperation
These annotations are used for integrating Swagger for API documentation.
@Configuration
@EnableSwagger2
public class SwaggerConfig {
// Swagger configuration
}
@RestController
@RequestMapping("/api/users")
@Api(tags = "User API")
public class UserController {
@GetMapping("/{userId}")
@ApiOperation("Get user by ID")
public ResponseEntity<User> getUserById(@PathVariable Long userId) {
// Retrieve user by ID and return as ResponseEntity
}
}
17. Environment and Property Annotations
@PropertySource
, @Value
These annotations are used for loading external properties into Spring environment variables.
@Configuration
@PropertySource("classpath:myapp.properties")
public class MyAppPropertiesConfig {
// Property configuration
}
@Service
public class MyService {
@Value("${myapp.api.url}")
private String apiUrl;
// Use apiUrl in service methods
}
18. Actuator Annotations
@Endpoint
, @ReadOperation
, @WriteOperation
These annotations are used for creating custom endpoints for Spring Boot Actuator.
@Endpoint(id = "custom")
public class CustomEndpoint {
@ReadOperation
public String getCustomInfo() {
// Read operation implementation
}
@WriteOperation
public void updateCustomInfo(String newInfo) {
// Write operation implementation
}
}
These Spring Boot annotations cover various aspects of application development, including transactions, messaging, internationalisation, documentation, property management, and actuator customization. Integrating these annotations into your Spring Boot projects will help you address specific concerns and enhance the functionality of your applications. The flexibility and extensibility provided by Spring Boot annotations contribute to its popularity among developers for building Java-based applications.
You can read the official Spring documentation here – Spring Docs
In the last BitsToGigs post we had discussed How to Create Custom Annotations in a Spring Boot App.
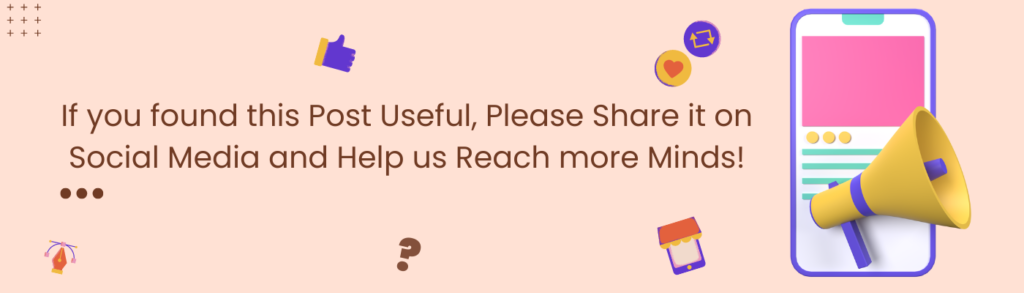
Happy Learning!
Subscribe to the BitsToGigs Newsletter