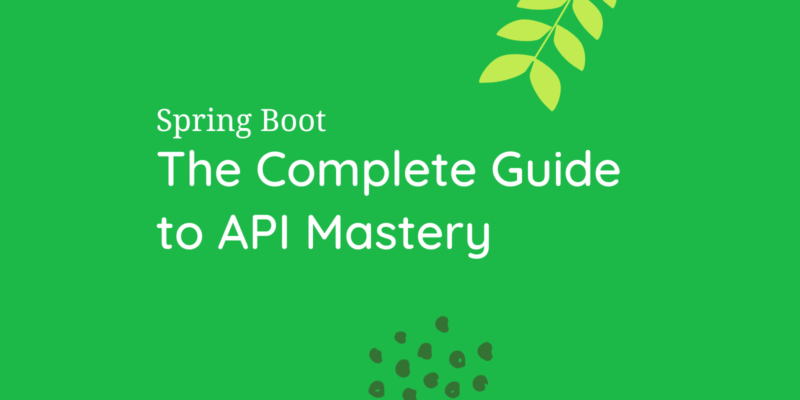
The Complete Guide to Spring Boot API Mastery
Spring Boot has become a cornerstone in the world of Java-based web application development, and its capabilities extend seamlessly to API development. In the Complete Guide to Spring Boot API Mastery post, we’ll delve into the art of crafting robust APIs using Spring Boot, exploring multiple examples, best practices, and low-level details that every Spring Boot developer should be acquainted with.
Understanding the Basics
1. Project Setup
Before diving into API development, it’s essential to set up a Spring Boot project. You can use the Spring Initializr to bootstrap your project with the necessary dependencies. Include “Spring Web” in your dependencies to leverage Spring’s web capabilities.
@SpringBootApplication
public class MyApiApplication {
public static void main(String[] args) {
SpringApplication.run(MyApiApplication.class, args);
}
}
2. RESTful Principles
Spring Boot promotes the development of RESTful APIs. Adhering to RESTful principles ensures a scalable and maintainable API. Key principles include resource identification, stateless communication, and a uniform interface.
Creating a Simple REST Controller
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/hello")
public String sayHello() {
return "Hello, World!";
}
}
In this example, the @RestController
annotation indicates that this class is a REST controller, and @RequestMapping
specifies the base path for all the endpoints in this controller.
Handling Requests and Responses
3. Request Mapping
Define API endpoints with @RequestMapping
, specifying HTTP methods, paths, and variables like path variables for dynamic data extraction.
@GetMapping("/greet/{name}")
public String greet(@PathVariable String name) {
return "Hello, " + name + "!";
}
Here, {name}
is a path variable that can be extracted from the URL.
4. Request Parameters
Handle query parameters using @RequestParam
, enabling the extraction of values from the URL for more flexible API behavior.
@GetMapping("/greet")
public String greetWithParam(@RequestParam String name) {
return "Hello, " + name + "!";
}
5. Request Body
Utilize @RequestBody
for receiving JSON or XML payloads in the request body, enabling the creation or update of resources.
@PostMapping("/user")
public ResponseEntity<String> createUser(@RequestBody User user) {
// Process user creation logic
return ResponseEntity.status(HttpStatus.CREATED).body("User created successfully");
}
Validation and Error Handling
6. Input Validation
Enhance API robustness by employing validation annotations like @Valid
and others to ensure the integrity of incoming data.
@PostMapping("/user")
public ResponseEntity<String> createUser(@Valid @RequestBody User user) {
// Process user creation logic
return ResponseEntity.status(HttpStatus.CREATED).body("User created successfully");
}
7. Global Exception Handling
Enabling global exception handling in Spring Boot is crucial for providing a unified approach to error management. By employing the @ControllerAdvice
annotation, you can create a central class to handle exceptions thrown across multiple controllers.
The @ExceptionHandler
method within this class allows customization of the response for specific exception types. This ensures consistency in error responses, making it easier for clients to understand and handle errors gracefully.
Global exception handling promotes code maintainability by consolidating error-handling logic in one location. It also enhances the overall user experience by presenting standardized error messages, aiding developers in identifying and resolving issues during development and troubleshooting.
Global exception handling in Spring Boot contributes to the resilience of your API, ensuring a more robust and user-friendly experience by gracefully handling unexpected situations.
Implement a global exception handler using @ControllerAdvice
to centralize error handling.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleException(Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(e.getMessage());
}
}
Data Persistence
8. JPA Integration
Integrate Java Persistence API (JPA) for seamless database operations, facilitating the mapping of Java objects to database entities.
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String email;
// Getters and setters
}
9. Repository Interface
Create a repository interface extending JpaRepository for standardized CRUD operations on your data entities.
public interface UserRepository extends JpaRepository<User, Long> {
// Additional query methods if needed
}
10. Service Layer
Implement a service layer to separate business logic from controller responsibilities, promoting cleaner and more maintainable code.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User createUser(User user) {
// Business logic
return userRepository.save(user);
}
}
Security
11. Securing Endpoints
Implementing security with Spring Security is vital to safeguard your API. By configuring access rules using authorizeRequests
, you can control which endpoints are accessible to different user roles. For instance, granting public access to certain endpoints while requiring authentication for more sensitive operations.
Incorporating a login mechanism with formLogin()
allows users to authenticate via a login page, enhancing the security of protected resources. Similarly, the logout()
configuration facilitates secure user logouts.
Spring Security’s flexibility extends to supporting various authentication mechanisms, including JWT, OAuth, and more. This adaptability allows developers to choose the authentication strategy that best aligns with their application’s security requirements.
In essence, securing endpoints in Spring Boot ensures that only authorized users can access protected resources, fortifying your API against unauthorized access and potential security threats.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/api/public/**").permitAll()
.antMatchers("/api/private/**").authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.logoutUrl("/logout")
.permitAll();
}
}
Documentation
12. API Documentation with Swagger
Integrating Swagger in your Spring Boot project allows for automatic and interactive API documentation. By configuring a Swagger Docket bean, you enable the generation of a dynamic API documentation UI. This UI provides developers with an interactive interface to explore your API’s endpoints, request/response formats, and even allows them to test API calls directly from the documentation.
Swagger annotations, such as @Api
, @ApiOperation
, and @ApiModel
, offer fine-grained control over the generated documentation, enabling you to provide meaningful descriptions, deprecate endpoints, and define data models. Additionally, Swagger simplifies collaboration by serving as a living document that stays in sync with your evolving API, reducing the likelihood of documentation becoming outdated.
By incorporating Swagger into your Spring Boot project, you enhance the discoverability and usability of your API, fostering a smoother development experience for both internal and external stakeholders.
@Configuration
@EnableSwagger2
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.api"))
.paths(PathSelectors.any())
.build();
}
}
Testing
13. Unit Testing
Ensure code reliability through unit testing with tools like JUnit and Mockito, verifying the functionality of individual components.
@RunWith(MockitoJUnitRunner.class)
public class UserControllerTest {
@Mock
private UserService userService;
@InjectMocks
private UserController userController;
@Test
public void testCreateUser() {
// Test logic
}
}
14. Integration Testing
Conduct integration tests using @SpringBootTest
to evaluate the behavior of your API as a whole in a controlled environment.
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
public class UserControllerIntegrationTest {
@Autowired
private TestRestTemplate restTemplate;
@Test
public void testCreateUser() {
// Test logic
}
}
Best Practices
15. Use DTOs
Decouple internal domain models from API contracts using Data Transfer Objects (DTOs), promoting flexibility and abstraction.
public class UserDto {
private String username;
private String email;
// Getters and setters
}
16. Pagination and Sorting
Implement pagination and sorting for resource-heavy APIs, ensuring efficient data retrieval and improved user experience.
@GetMapping("/users")
public Page<User> getUsers(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size,
@RequestParam(defaultValue = "id,asc") String[] sort) {
return userService.getUsers(PageRequest.of(page, size, Sort.by(sort)));
}
17. Versioning
Consider versioning your API, either through URI versioning or request headers, to manage and communicate changes effectively over time.
@RestController
@RequestMapping("/api/v1/users")
public class UserControllerV1 {
// Controller logic
}
@RestController
@RequestMapping("/api/v2/users")
public class UserControllerV2 {
// Updated controller logic
}
You can read more in detail on Spring Boot website Spring Boot
Mastering API development in Spring Boot involves a deep understanding of its various features and best practices. From handling requests and responses to securing your endpoints and documenting your API, each step contributes to building a robust and maintainable system. By following these examples and incorporating these best practices, Spring Boot developers can create APIs that meet the highest standards of quality and reliability.
In our last BitsToGigs.com post we had discussed about cypress tests. You can learn more about here – All FrontEnd Developers need to Know about Cypress Tests
Happy Learning!
Subscribe to the BitsToGigs Newsletter