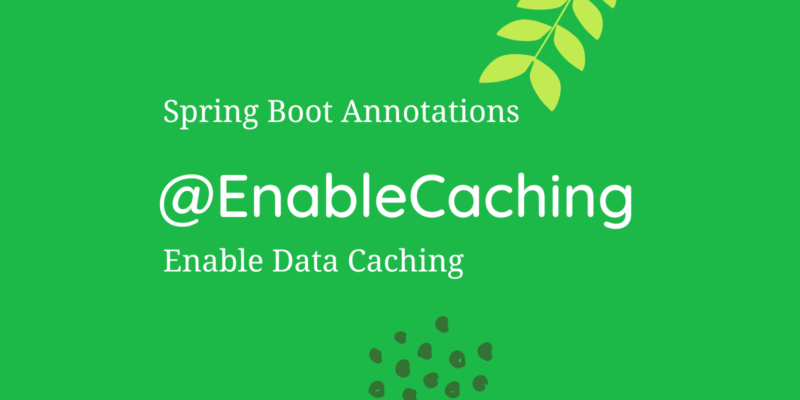
How to use Spring Boot @EnableCaching Annotation for Data Caching
Caching is a technique to store frequently used data in memory or on disk, in order to reduce the time it takes to retrieve the data from the database or external services. Caching is important for improving the performance of web applications, as it reduces the time required to retrieve data, thus reducing the response time of the application. Spring Boot provides caching support through its @EnableCaching
Annotation for Data Caching.
@EnableCaching Annotation
The @EnableCaching
annotation is used to enable caching in a Spring Boot application. This annotation is used on the application’s main configuration class, which is typically annotated with @SpringBootApplication
. The @EnableCaching
annotation allows Spring Boot to automatically configure the caching infrastructure based on the dependencies available in the classpath.
Let’s take a look at the uses of the @EnableCaching
annotation in the following examples.
Example 1
Let’s consider a simple example to understand the usage of the @EnableCaching
annotation. Suppose we have a Spring Boot application that retrieves customer data from a database. The CustomerService
class retrieves customer data from the database and has a method to retrieve a customer by their ID.
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
public Customer getCustomerById(Long id) {
return customerRepository.findById(id).orElse(null);
}
}
JavaTo enable caching for the getCustomerById()
method, we need to add the @Cacheable
annotation to it. The @Cacheable
annotation tells Spring to cache the result of the method for future requests with the same arguments.
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
@Cacheable(value = "customers", key = "#id")
public Customer getCustomerById(Long id) {
return customerRepository.findById(id).orElse(null);
}
}
In the above code, the @Cacheable
annotation specifies the cache name as customers
and the cache key as the customer ID. This means that if a request is made with the same customer ID, the result will be fetched from the cache instead of the database.
To enable caching in the application, we need to add the @EnableCaching
annotation to the main configuration class.
@SpringBootApplication
@EnableCaching
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In the above code, the @EnableCaching
annotation enables caching in the application.
Example 2
Now let’s consider a more complex example where we have multiple cache providers and want to configure them in the application. Suppose we have a Spring Boot application that retrieves customer data from multiple data sources, such as a database and a REST API. We want to cache the data retrieved from each data source separately. To achieve this, we need to configure multiple cache managers in the application.
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
SimpleCacheManager cacheManager = new SimpleCacheManager();
List<Cache> caches = new ArrayList<>();
caches.add(new ConcurrentMapCache("databaseCache"));
caches.add(new ConcurrentMapCache("apiCache"));
cacheManager.setCaches(caches);
return cacheManager;
}
}
In the above code, we have created a CacheConfig
class that configures the cache managers for the application. The cacheManager()
method creates a SimpleCacheManager
and adds two ConcurrentMapCache
instances to it, one for each data source
To enable caching in the application, we need to add the @EnableCaching
annotation to the main configuration class.
@SpringBootApplication
@EnableCaching
@Import(CacheConfig.class)
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
In the above code, we have imported the CacheConfig
class using the @Import
annotation. This allows us to use the cache managers defined in the CacheConfig
class in our application.
Now let’s modify the CustomerService
class to retrieve data from both the database and the REST API.
@Service
public class CustomerService {
@Autowired
private CustomerRepository customerRepository;
@Autowired
private RestTemplate restTemplate;
@Cacheable(value = "databaseCache", key = "#id")
public Customer getCustomerByIdFromDatabase(Long id) {
return customerRepository.findById(id).orElse(null);
}
@Cacheable(value = "apiCache", key = "#id")
public Customer getCustomerByIdFromApi(Long id) {
String url = "https://api.example.com/customers/" + id;
ResponseEntity<Customer> response = restTemplate.getForEntity(url, Customer.class);
return response.getBody();
}
}
In the above code, we have added two methods to retrieve customer data from the database and the REST API. We have also added the @Cacheable
annotation to each method to enable caching.
To use the CustomerService
class in our application, we can create a REST endpoint to retrieve customer data by their ID.
@RestController
public class CustomerController {
@Autowired
private CustomerService customerService;
@GetMapping("/customers/{id}")
public ResponseEntity<Customer> getCustomerById(@PathVariable Long id) {
Customer customer = customerService.getCustomerByIdFromDatabase(id);
if (customer == null) {
customer = customerService.getCustomerByIdFromApi(id);
}
if (customer == null) {
return ResponseEntity.notFound().build();
}
return ResponseEntity.ok(customer);
}
}
In the above code, we have created a REST endpoint to retrieve customer data by their ID. We have also used the CustomerService
class to retrieve the data from the database and the REST API. If the data is not found in the database, we retrieve it from the REST API. If the data is still not found, we return a 404 Not Found
response.
The @EnableCaching
annotation in Spring Boot allows us to easily enable caching in our application. By using the @Cacheable
annotation, we can cache the results of frequently used methods, which improves the performance of our application. The complex example shows how we can configure multiple cache providers in the application and use them to cache data from multiple data sources.
In the last post we had discussed about A Practical Guide to Kafka Integration with Spring Boot. You can read that post here.
Happy Learning! Please follow us on Twitter.
Subscribe to the BitsToGigs Newsletter